Ways to run Code on Application Startup in Spring Boot
Let’s learn how to run a piece of code at the startup of a spring boot application.
Using CommandLineRunner or ApplicationRunner interface
You could provide a Bean of type CommandLineRunner or an ApplicationRunner interface. The perk of using this approach allows your code to have access to the application arguments.
@Bean
CommandLineRunner commandLineRunner() {
return new CommandLineRunner() {
@Override
public void run(String... args) throws Exception {
log.info("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~");
log.info("These Lines will get printed on application startup");
log.info("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~");
}
};
}
Code language: JavaScript (javascript)
or
@Bean
ApplicationRunner applicationRunner() {
return new ApplicationRunner() {
@Override
public void run(ApplicationArguments args) throws Exception {
log.info("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~");
log.info("These Lines will get printed on application startup too");
log.info("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~");
}
};
}
Code language: PHP (php)
Note that the only difference between these snippets is that you get more control over the parameters through the ApplicationArguments object.
Using Event Listeners to run Code on Application Startup
Spring boot emits a lot of events throughout its application lifecycle. So, we can define listeners for an ApplicationStartedEvent or a ContextRefreshedEvent to know when to run our code.
@EventListener
public void executeOnStartup(ApplicationStartedEvent event) {
log.info("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~");
log.info("Another way to run something on application startup with event {}", event);
log.info("~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~");
}
Code language: CSS (css)
Most importantly, you could access the application context through the event parameter.
To run your code after the context is complete but before the application is started, Then you should use the ContextRefreshedEvent.
Through SmartInitializingSingleton
The last and probably the simpler approach is to define an implementation for SmartInitializingSingleton. Spring boot looks for this call-back bean after all the singleton bean creations are complete.
@Bean
SmartInitializingSingleton smartInitializingSingleton(ApplicationContext context) {
return () -> {
log.info("This runs on startup too... {}", context);
};
}
Code language: JavaScript (javascript)
This approach is helpful when you have a simple scenario and don’t want to include any dependencies other than the spring-beans jar.
Also, this implementation is somewhat synonymous with a listener of ContextRefreshedEvent.
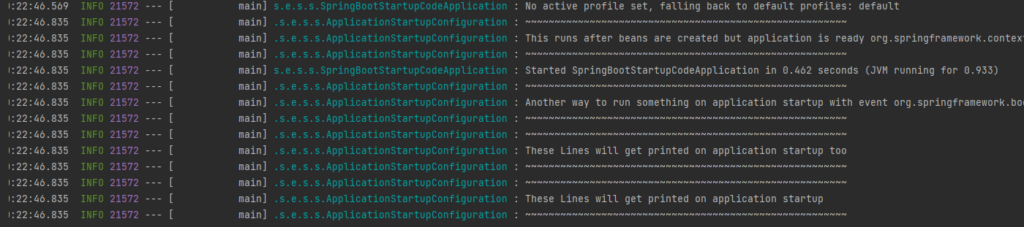
You could find all these examples to run code on startup in a spring boot application at this gist.