Changing Context Path in a Spring Boot Application
By default, Spring boot has “/” as the context path. If you wish to override/change the context path, then you can use one of the following approaches.
In most scenarios, the default context path is all you would want. It gives a clean approach to writing APIs.
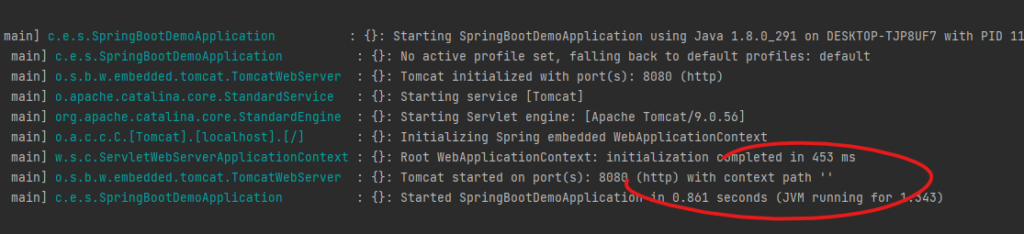
However, there are some cases where you might want to use your application under a different context path. They are,
- You are migrating from treditional deployment where all web applications had a context path (eg. Standalone tomcat WAR deployments)
- You want to use same load balancer for different applications with same API paths.
- Having different versions of the same app. (Apps with APIs starting with /v1, /v2 etc).
In this post, We will explore the ways to change the default context path of a spring boot application.
Using Configuration properties
Spring boot provides an easy way to override the context via the “server.servlet.context-path” property. For example, the below sets the context path to /springhow.
server.servlet.context-path=/springhow
Code language: Properties (properties)
And if you are using YAML, then the following is the way to do it.
server:
servlet:
context-path: /springhow
Code language: YAML (yaml)
Furthermore, Spring boot lets you pass properties through the JVM parameter.
java -Dserver.servlet.context-path=/springhow -jar hello-world-jar
Code language: Java (java)
Also, you can pass this configuration as a program parameter.
java -jar hello-world-jar --server.servlet.context-path=/springhow
Code language: Java (java)
Spring Boot also takes OS variables for config. If you wish to use this, then you need to match the environment variable with the name of the config. For windows, you can use the following commands.
set SERVER_SERVLET_CONTEXT_PATH=/springhow
java -jar hello-world-jar
Code language: JavaScript (javascript)
And for Linux and mac, You need to set the environment variable using the export
command.
export SERVER_SERVLET_CONTEXT_PATH=/springhow
java -jar hello-world-jar
If you are using the Spring boot 1.X version, then you should use the server.context-path for properties. and SERVER_CONTEXT_PATH for the environment variable.
Programatically changing the context path in Spring Boot
There are a couple of ways to programmatically change the context path. The first and most simple one is to set the “server.servlet.context-path” as a system property.
@SpringBootApplication
public class SpringBootDemoApplication {
public static void main(String[] args) {
System.setProperty("server.servlet.context-path","/springhow");
SpringApplication.run(SpringBootDemoApplication.class, args);
}
}
Code language: PHP (php)
The WebServerFactoryCustomizer interface lets you customize the underlying embedded server container. By providing a bean implementation for this interface, You can customize the webserver. In our case, we are overriding the default context path.
@Bean
WebServerFactoryCustomizer<ConfigurableServletWebServerFactory> webServerFactoryCustomizer() {
return factory -> {
factory.setContextPath("/springhow");
};
}
Code language: JavaScript (javascript)
You could also change the application port, session behavior, etc using this implementation.
With proper settings, you can see the results in the logs.
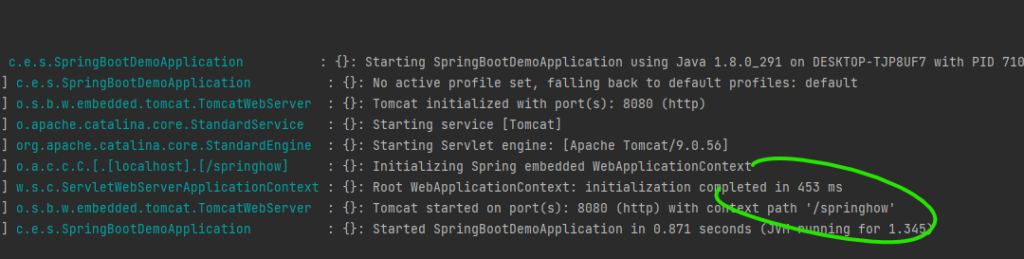
Summary
We learned various ways to change the context path of a spring boot application. Note that the programmatic approach is there to provide a permanent and/or dynamic solution. So always prefer to config if you plan on changing the context path often.