Accessing application.properties in Spring Boot
In this post, We will take a look at accessing property values from the application.properties in a spring boot application with examples.
Using @Value annotation
The easiest way to access values from application.properties or application.yaml is to use the @Value annotation. Just define these annotations in your Bean classes and spring will populate the values for you.
@Value("${app.greeting.message}")
private String greetingMessage;
Code language: CSS (css)
The @Value annotation casts the properties values into their appropriate field types. You can learn more about @Value at A guide to @Value in Spring Boot.
Using @ConfigurationProperties
The @ConfigurationProperties annotation lets you create POJO beans based on the configuration properties.
Firstly, you need to group a list of properties entries with some prefix. For example, here are some properties that we would like to access in a structured manner.
app.greeting=Hello People...!
app.api-endpoint=https://api.example.com/v1
Code language: JavaScript (javascript)
After that, you need to configure your bean to have @Configuration properties like in the below example.
@Configuration @ConfigurationProperties(prefix = "app") public class AppConfig { private String greeting; private String apiEndpoint; //getter setter }
Note that the @ConfigurationProperties annotation has a prefix value. This narrows down which entries to match against the POJO fields. You can also define property keys with hyphens to match their field names for readability.
In addition to that, let’s write a simple controller to print the output.
@Autowired
AppConfig appConfig;
@RequestMapping("/test")
@ResponseBody
private AppConfig getAppConfig() {
return appConfig;
}
Code language: CSS (css)
As you see below, the values reflect properly.
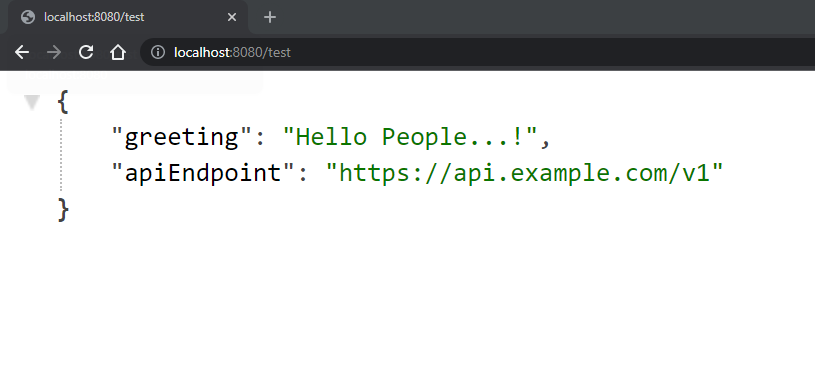
Furthermore, You could create more fields within this class and autowire the bean wherever you want. This way, You don’t need to write the same @Value annotation everywhere.
Using Environment Bean to access application.properties
This approach is rather counterintuitive. However, you could get any value by key from the application.properties using the Environment bean from spring.
@Autowired
Environment environment;
private String getAppConfigFromEnv() {
return environment.getProperty("app.greeting");
}
Code language: JavaScript (javascript)
With this approach, you get to access all values manually. And you lose the automatic validations from POJO and the ease of @Value annotations.
Summary
To sum up, the best way to access the application.properties values in spring boot are to load them through @ConfigurationProperties. Never use environment.getProperty() unless it’s absolutely necessary. The @Value annotation can help if there are only a few entries.
Related
Sample projects are available at github.com/springhow.