Decorator Design Pattern
Decorator Design pattern allows us to add behaviour to an object without affecting the other objects of the same class.
When to use the decorator pattern
If you want an object to gain (or lose) functionality at runtime, then you should use the decorator pattern. This approach lets us add features to a class without having to extend it.
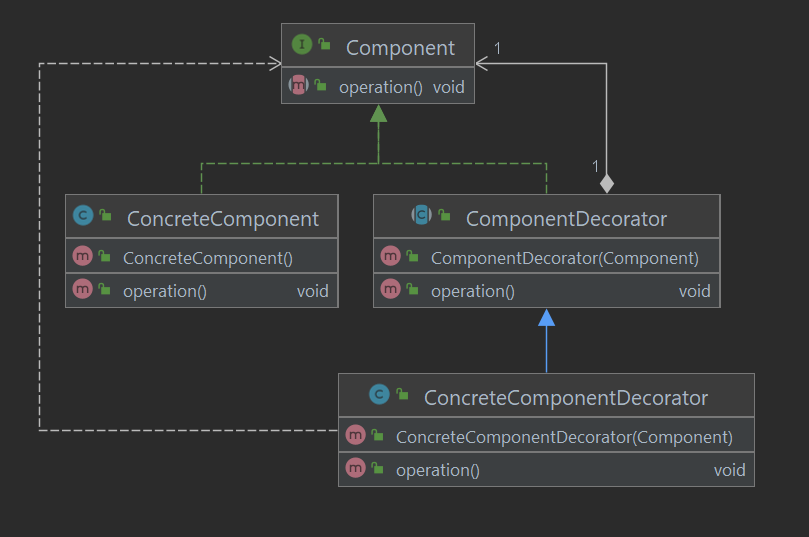
To simply put, The Decorator Design Pattern attaches additional responsibilities to an object dynamically. Decorators provide a flexible alternative to subclassing for extending functionality
Implementation
As you see from the UML diagram, We first need to identify our components. For example, I’m using the Car
interface. With this, We could provide a concrete implementation, say SimpleCar
.
public interface Car {
String drive();
}
Code language: PHP (php)
public class SimpleCar implements Car {
@Override
public String drive() {
return "Drive!";
}
}
Code language: PHP (php)
Now we have a SimpleCar
that can drive. But now, we need to build a sports car with added features. As the simple car can already be tuned into a sports car, We are going to use a decorator to help solve this issue.
Decorator Java class
Here is the decorator class for the simple car. This class is abstract as it can only solve its purpose when you extend it.
public abstract class SimpleCarDecorator implements Car {
protected SimpleCar car;
public SimpleCarDecorator(SimpleCar car) {
this.car = car;
}
public String drive() {
return car.drive();
}
}
Code language: PHP (php)
As we have an abstract version, let’s implement it to provide a Sports Car version.
package com.springhow.designpatterns.decorator;
public class SportsCardDecorator extends SimpleCarDecorator {
public SportsCardDecorator(SimpleCar car) {
super(car);
}
@Override
public String drive() {
return super.drive() + " Faster...!";
}
public void tunePerformance(){
System.out.println(" performance tuned..!");
}
}
Code language: JavaScript (javascript)
The important things to note here is that the drive method has a new implementation. It represents a faster car. Also , the class provides extra features for tuning.
Advantages and disadvantages of Decorator Pattern
Like other design patterns, The decorator design pattern also has its merits and disadvantages. Let’s go through each of them in detail.
Advantages | Disadvantages |
---|---|
It Lets you add functionality without subtyping a given class. | The clients may not know if they are using an actual component implementation or a decorator. This may not necessarily a problem. But when they start extending decorator class instead of the components, then the code will become messy. |
With the decorator design pattern, You could switch to different features on the fly. You just have to choose the right decorator. |
You can find all these examples in our GitHub repository.