Facade Design Pattern
The facade design pattern is a structural design pattern used commonly in object oriented languages like Java. A facade is an object that serves as a front-facing interface masking more complex underlying or structural code.
the facade design pattern can:
- improve the readability and usability by masking interaction with more complex components behind a simplified API.
- provide a context-specific interface to more generic functionality.
- Help refactor tightly coupled systems to more loosely coupled code.
Implementing Facade Design Pattern in Java
For example, take a look at a service desk taking care of orders. In this example, you cannot process the order if the stock is unavailable or the payment is missing. But these conditions are too complex and sensitive. And the clients cannot take care of these themselves.
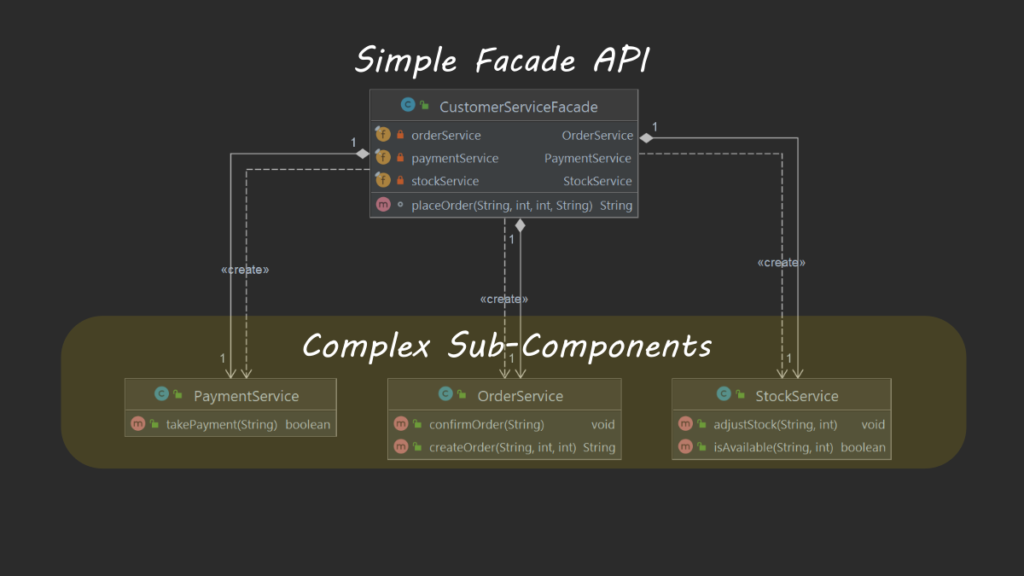
This is where a new Customer Service Facade comes into picture. It acts as a front with easy APIs to place or cancel an order. So lets implement this design pattern in java.
PaymentService
For this dummy service, We are checking if the card is 16 digits long. This service will be used by our facade pattern.
public class PaymentService {
public boolean takePayment(String cardDetails) {
return cardDetails.length() == 16;
}
}
Code language: Java (java)
Stock Service
This service returns true if the product in question contains the letter “s”. For example, “shirt” and “shoe” would result in true, but “tie” would result in false.
public class StockService {
public boolean isAvailable(String product, int count) {
if (product.contains("s")) {
System.out.println("The product is available");
return true;
}
System.out.println("The product is not available");
return false;
}
public void adjustStock(String product, int count) {
System.out.println("Product [" + product + "] is reduced by [" + count + "]");
}
}
Code language: Java (java)
all of these are to simulate our complex sub systems. In reality, A stock lookup would be large software dealing with inventories and third-party systems.
Order Service
Finally, we have an order service that creates and maintains orders.
package com.springhow.designpatterns.facade;
import java.util.Random;
import java.util.UUID;
public class OrderService {
public String createOrder(String product, int price, int count) {
System.out.println("Created an order for "+product+" x "+count+" for price : "+ price);
return UUID.randomUUID().toString();
}
public void confirmOrder(String orderNum) {
System.out.println("Order [" + orderNum + "] confirmed..!");
}
}
Code language: Java (java)
This will be our final mock service to demonstrate the facade design pattern in java.
Facade Class
Now that we have all classes, lets write our facade. As we understand already, facade is supposed to be a simple and beautiful API. It is supposed to hide all the internal logics and only provide what is necessary for the client.
public class CustomerServiceFacade {
private final OrderService orderService = new OrderService();
private final PaymentService paymentService = new PaymentService();
private final StockService stockService = new StockService();
String placeOrder(String product, int count, int price, String cardDetails) {
boolean stockAvailable = stockService.isAvailable(product, count);
String orderNum;
if (stockAvailable) {
orderNum = orderService.createOrder(product, price, count);
} else {
throw new RuntimeException("Stock Not available");
}
boolean success = paymentService.takePayment(cardDetails);
if (success) {
orderService.confirmOrder(orderNum);
stockService.adjustStock(product, count);
} else {
throw new RuntimeException("Payment Failed");
}
return orderNum;
}
}
Code language: Java (java)
As you see, All the services are private and are not available for the client classes. And, this class exposes a single method for order processing. If the client had to do this, then it would be fairly difficult. This is a classic example of a facade pattern.
A client that uses facade pattern
Finally, A client in design pattern can be any class or library that utilizes that pattern. For example, the below snippet uses the “customerServiceFacade” java object which is an example of a facade design pattern.
public class Client {
public static void main(String[] args) {
CustomerServiceFacade customerServiceFacade = new CustomerServiceFacade();
String orderNum = customerServiceFacade.placeOrder("shirt", 2, 100, "1234567890123456");
System.out.println("Order Num = " + orderNum);
}
}
Code language: Java (java)
Advantages of Facade Design pattern
Facade helps you simply deal with complex libraries. How complex the implementation may be, If we could identify certain common problems that libraries solve, then we can expose these solutions through facade pattern methods. These methods will become API entry points for the entire library, thus making the client libraries’ life easier.
You can find these examples in our GitHub repository.