Design Patterns in Java
In software engineering, Design patterns in java are the solution to write better applications. They are the best practices to solve certain problems in various situations. And These patterns are not algorithms. And they are definitely not a programming paradigm. But, it is just an approach to building concrete programs.
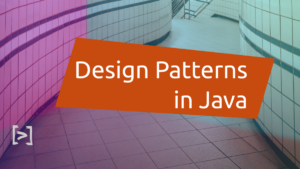
In 1994 Erich Gamma, Richard Helm, Ralph Johnson and John Vlissides published the book Design Patterns: Elements of Reusable Object-Oriented Software. We now know these authors as the Gang of Four (GoF).
This book goes on to explain various approaches to object-oriented programming. In those approaches, two instructions stand out. They are,
- Always program to interfaces, not implementation.
- Do not reuse code through inheritance (object composition over inheritance).
In this series of tutorials, We will go through each java design pattern in detail.
Types of Design patterns in Java
As per the Gang of four(GOF) there are 23 patterns. And we can classify them in to the following 3 types.
Creational Patterns
To begin with, The Creational patterns deal with the object creation based on the situation. When basic object creation causes added complexity to the design, these patterns deal with it by controlling the object creation.
Some well known creational patterns are,
Structural Design Patterns
Further, We have the Structural patterns take care of class and object composition. These patterns use inheritance to create proxies and adapters that allow one class to used as another interface. Some important patterns in this category are,
- Adapter pattern
- Bridge pattern
- Composite pattern
- Decorator pattern
- Facade pattern
- Proxy Pattern
- Flyweight pattern
Behavioral Patterns
At last, We have the Behavioral Patterns that deal with communication between objects and their behaviour in the system. They provide approach to adding new features.
- Command pattern
- Interpreter pattern
- Chain of Responsibility pattern
- Iterator pattern
- Mediator pattern
- Strategy pattern
- Memento pattern
- Observer pattern
- State pattern
- Visitor pattern
- Template method Pattern
Why use design patterns?
Let’s go through the reasons why you should use design patterns in your java applications.
Don’t reinvent the wheel
Design patterns are hard earned experiences. That is, There is almost always a design pattern to solve a design problem. So it is easier for developers to find the right solution for a problem
Fewer Changes
All software development projects are susceptible to change. That is, As changes directly correlate with amount of effort and money, It makes sense to design the system that changes very little. Most of the behavioural patterns help deal with changes in the project. These patterns can introduce new behaviours to the project without huge effort from the developers.
Recognizable code
Once you master the design patterns, you can easily understand anyone else’s code(if they use design patterns). This advantage is crucial when the developers need to work with third party libraries. After all, the best documentation for the code is the code itself. To conclude, the patterns help you understand and write better code.