Form Login with Spring Boot
This article concentrates on the default form login implementation from Spring Boot and Spring Security. Let’s dive in to understand spring security with form-based username and password login.
To start with, I have written a simple web application with an API that prints hello world.
@RestController
public class HelloController {
@RequestMapping("/hello")
@ResponseBody
public String hello() {
return "Hello World!";
}
}
Code language: PHP (php)
There is nothing special about this Controller. When we open http://localhost:8080/hello from the browser, we get to see a ”Hello World!” message.
Adding Spring Security Starter
Let’s add the spring security module to this project by adding the following starter dependency.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
Code language: HTML, XML (xml)
Once you have added the above dependency, Restart the application and try accessing http://localhost:8080/hello again in the browser. This time you will see a form login shown by the spring boot application
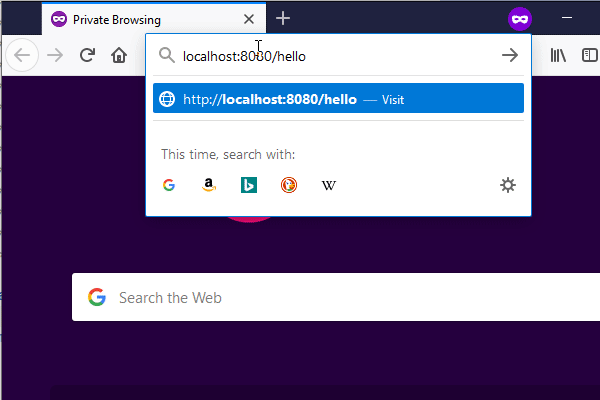
This behavior is due to the magic of auto-configuration. Let’s dive into details.
Security auto-configuration
This starter for security does the following auto-configurations.
- Creates a servlet
Filter
calledspringSecurityFilterChain
which is responsible for most of the security stuff like login form redirection, username password validation, session creation and destruction etc. ThisspringSecurityFilterChain
will be registered to intercept all URLs of your application. - Sets up BasicAuth and a form login for all the requests. This setup is equivalent to the following.
protected void configure(HttpSecurity http) throws Exception { http.authorizeRequests() .anyRequest().authenticated() .and().formLogin() .and().httpBasic(); }
We will see these builder methods in upcoming posts. - Creates a simple in-memory
UserDetailService
that can only allow a username calleduser
with a generated password. You can find this password in the startup logs.
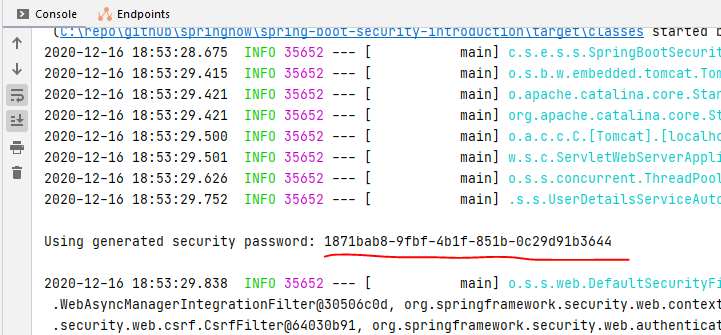
By default, Spring Security enables a logout endpoint at http://localhost:8080/logout. On opening this URL, You will see a logout button. And By clicking this button You can log out of the session. Subsequently, the application will redirect you to a login screen.
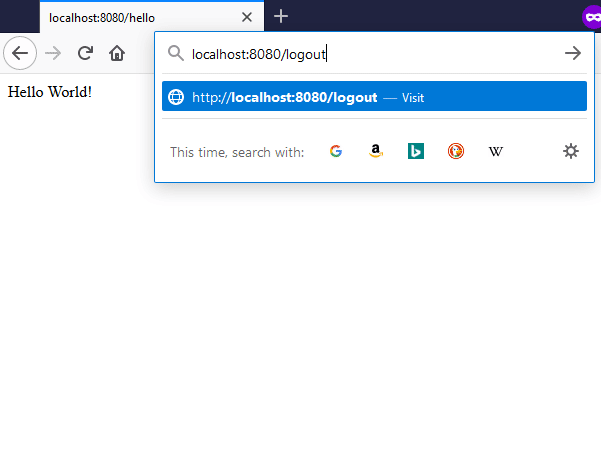
Login flow
Here is a simple illustration of the form login works.
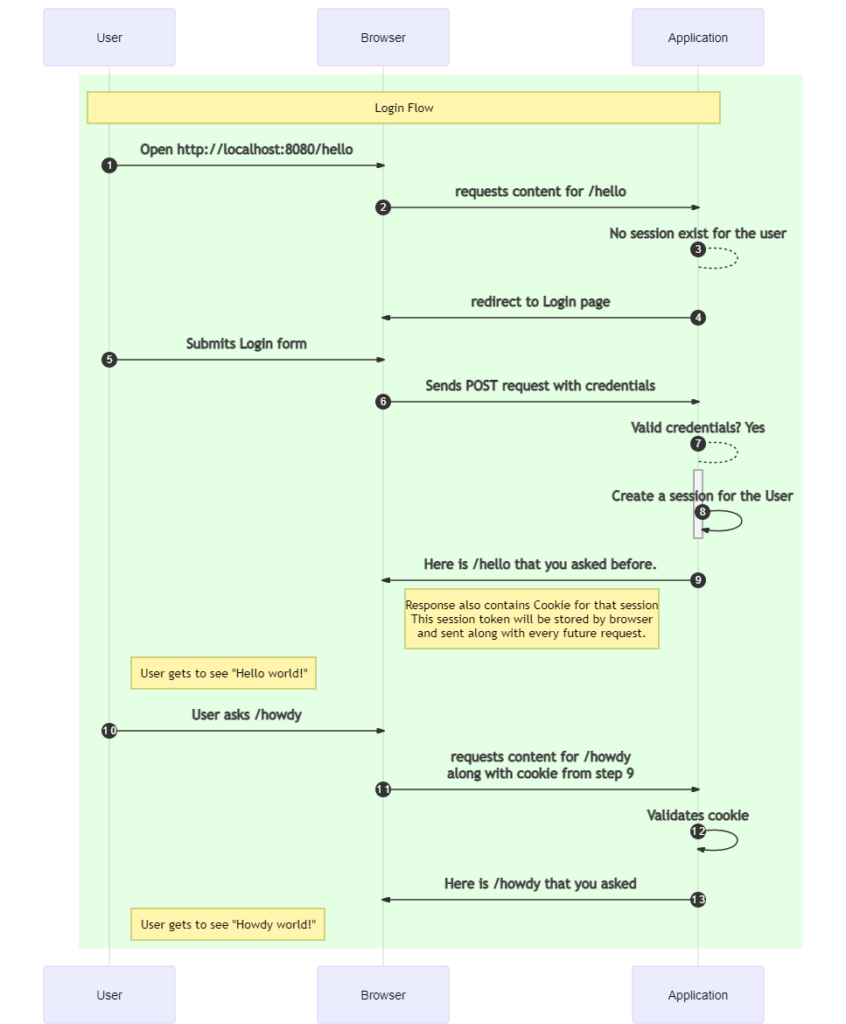
To understand the flow, you need to learn a little about cookies.
- Cookies are key-value pair with occasional expiry time associated with it.
- Cookies are created when the server/website/web-application sends an HTTP response with a Set-Cookie header.
- By design, All browsers will send cookies associated with the website when accessing any resource from that specific server/website/web-application.
- Websites cannot get hold of cookies from other websites.
When a login request comes to the server, the spring security logic validates the credentials and if successful, creates a session id and associates it with the logged-in user, and keeps this mapping somewhere. The same session-id also sent back to the browser in the form of Set-Cookie
 header with the name JSESSIONID
.
Now whenever the request goes to the server, the cookie will also be sent along with the request. When the server notices JSESSIONID
, it can look at the session map and find the appropriate user associated with that session. If no mappings are found, the request will be redirected to a log-in page.
Logout flow
Here is the logout flow for simple Spring boot form implementation.
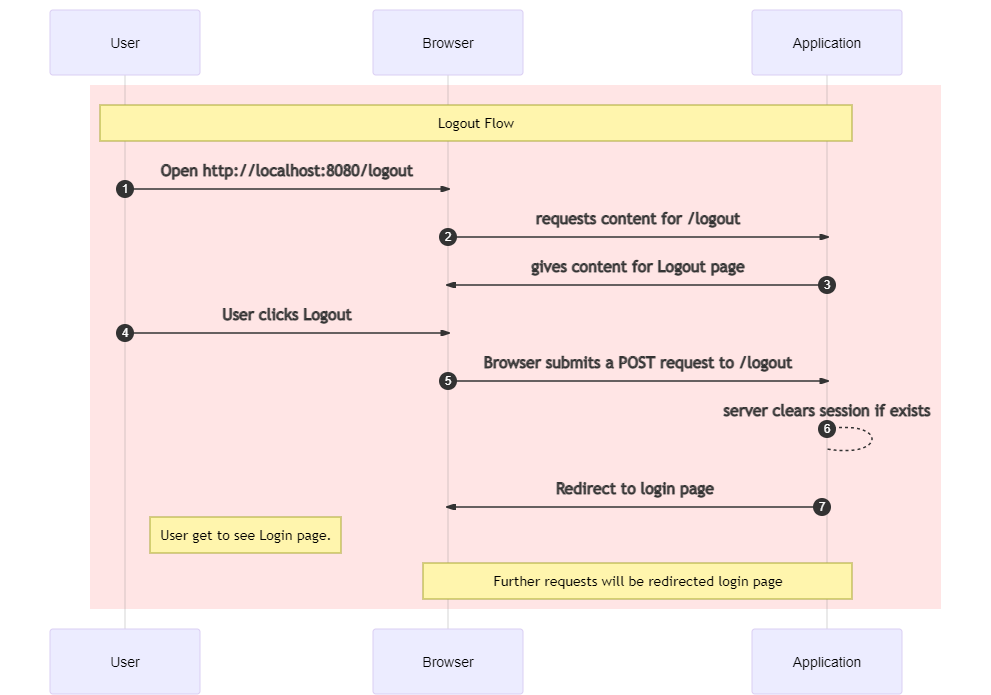
Up next, learn about the custom login form in Spring Boot.