Boolean in Thymeleaf for Conditional Evaluation
Thymeleaf relies a lot on boolean conditionals to render the HTML views. In this post, we will see how thymeleaf handles boolean values using th:if and th:unless directives.
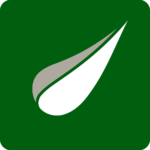
Boolean expressions in Thymeleaf
In Thymeleaf, all expressions can act as a Boolean expression. For example, The following values are considered false.
- An expression or a literal that is false.
- A String literal that equals to any one of false, no and off.
- A null object, or the null literal.
- The number literal, or an expression that equals to number 0.
- The NUL character U+0000.
Everything except these values are considered true in thymeleaf expressions.
Conditional Rendering
Thymeleaf relies on two attributes to render elements conditionally, and they are th:if
and th:unless
. Both of these attributes take a boolean expression and try to render as their names suggest.
That is, The thymeleaf th:if
attribute renders the element only if the boolean condition is true
. The th:unless
attribute does the exact opposite. To explain, here are some examples to help you understand these attributes.
<div th:if="${true}">This Element will be visible</div>
<div th:if="${false}">This Element will not be visible</div>
<div th:unless="${true}">This Element will not be visible</div>
<div th:unless="${false}">This Element will be visible</div>
Code language: HTML, XML (xml)
Boolean operations
Thymeleaf provides and
, or
and not
keywords to provide logical operations on Boolean expressions. These boolean operations in thymeleaf can be placed anywhere inside a variable expression. This way, we can combine and evaluate multiple expressions into one.
For example, The following example demonstrates how you can use the boolean operators in SpEL(Spring Expression language).
<nav>
<a href="/admin" th:if="${isLoggedIn and isAdmin}">Admin Console</a>
<a href="/reports" th:if="${isLoggedIn and (isUser or isAdmin})">View Reports</a>
<a href="/login" th:if="${not isLoggedIn}">Login</a>
<a href="/logout" th:if="${isLoggedIn}">Logout</a>
</nav>
Code language: HTML, XML (xml)
In the above example, SpEL takes care of expression evaluation. So, If you really want thymeleaf to take care of the expressions, you need to specify the keywords outside of ${…}. For instance, the above example can be written as the following.
<nav>
<a href="/admin" th:if="${isLoggedIn} and ${isAdmin}">Admin Console</a>
<a href="/reports" th:if="${isLoggedIn} and (${isUser} or ${isAdmin})">View Reports</a>
<a href="/login" th:if="not ${isLoggedIn}">Login</a>
<a href="/logout" th:if="${isLoggedIn}">Logout</a>
</nav>
Code language: HTML, XML (xml)
If you want to nest the boolean expressions you need to wrap them using braces as shown in the above examples.
Ternary and default operators For Boolean
The th:if
 and th:unless
 are the thymeleaf equivalent of if-else that take care of boolean expressions. So, You need some way to work with boolean within an expression level. For this reason, thymeleaf supports ternary expressions where you can specify if-then-else expressions. The syntax for this expression is similar to the one in java or C language. So, Here is an example that simplifies the previous snippet.
<a th:href="${isLoggedIn}? '/logout' : '/login'" th:text="${isLoggedIn} ? 'Logout' : 'Login'"></a>
Code language: HTML, XML (xml)
Sometimes, this ternary operator can even exclude the false criteria. For instance, the following is also possible.
<input class="${formInvalid} : text-red" type="text"/>
Code language: JavaScript (javascript)
Summary
To conclude, We learned how to make use of boolean better in a Thymeleaf environment. Furthermore, Take a look at Thymeleaf expressions and how to write a simple crud application with thymeleaf.
You could also Checkout our github repository for more examples on thymeleaf.