Handling Lists in Thymeleaf view
In this article, We will see how to handle Lists in thymeleaf templates with an example using th:each attribute.
Loop through a Lists
Let’s create a list of Objects first and then supply it to the Model and View. For this reason, We created a UserInfo
object.
public class UserInfo {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
private String firstName;
private String lastName;
}
Code language: Java (java)
The list we are going to use in our thymeleaf model is from database records. For this reason, We created the following service.
public List<UserInfo> getUsers() {
return userInfoRepository.findAllByActiveOrderByIdDesc(true);
}
Code language: Java (java)
Now that we have a list of UserInfo objects, Let’s write a controller method and map it to a thymeleaf view. In this case, the view name is “home.html”
@RequestMapping(path = "/", method = RequestMethod.GET)
public String getUsers(Model model) {
List<UserInfo> users = userService.getUsers();
model.addAttribute("userList", users);
return "home";
}
Code language: Java (java)
Here the list is called userList, and we can use th:each to loop through this list in the home.html
thymeleaf template as shown below.
<table>
<tr>
<th>ID</th>
<th>First Name</th>
<th>Last Name</th>
</tr>
<tr th:each="user : ${userList}">
<td class="align-middle" th:text="${user.id}"></td>
<td class="align-middle" th:text="${user.firstName}"></td>
<td class="align-middle" th:text="${user.lastName}"></td>
</tr>
</table>
Code language: HTML, XML (xml)
Empty lists in Thymeleaf View
In the above example, the table would look unpleasant if there are no rows. To handle these cases, You can make use the List.isEmpty()
method along with boolean expressions. Here is how you can do that.
<tr th:if="${userList.isEmpty()}">
<td class="text-center" colspan="3">No Records found. Add some...!</td>
</tr>
Code language: HTML, XML (xml)
You can also use the following expressions in the place of ${userList.isEmpty()}
.
${userList.empty}
– A short form of the above as isEmpty() is a getter for the field empty#lists.isEmpty(users)
– This expression used the built-in #lists utility object from thymeleaf.
Accessing list elements by position
If you want to display only the Nth element of a list, you can use any of the following methods to help yourself.
<div th:text="${users.get(2).firstName}"></div>
<div th:text="${users[2].firstName}"></div>
Code language: HTML, XML (xml)
Here, the first method uses typical java methods from a List
object.The latter uses SpEL to get the second item from a collection.
To get the first element of thymeleaf, you could use the following syntax.
<div th:text="${users.get(0).firstName}"></div>
<div th:text="${users[0].firstName}"></div>
Code language: HTML, XML (xml)
It is a bit difficult to get the last element straightaway. But it is still possible through.
<div th:text="${users.get(users.size()-1).firstName}"></div>
<div th:text="${users[users.size()-1].firstName}"></div>
Code language: HTML, XML (xml)
For Array objects you may need to use array.length
instead of list.size()
.
Loop information of List
With the above examples, there seems to be no way to get the current index of the elements. However, thymeleaf has something for this too. With th:each, you can also get a handle to a stats object that provides some crucial information about the iteration.
To access the stats variable, you need to rewrite your th:each
expression as shown here.
<tr th:each="user, stat : ${userList}">
<td class="align-middle" th:text="${stats.count}"></td>
<td class="align-middle" th:text="${user.id}"></td>
<td class="align-middle" th:text="${user.firstName}"></td>
<td class="align-middle" th:text="${user.lastName}"></td>
</tr>
Code language: HTML, XML (xml)
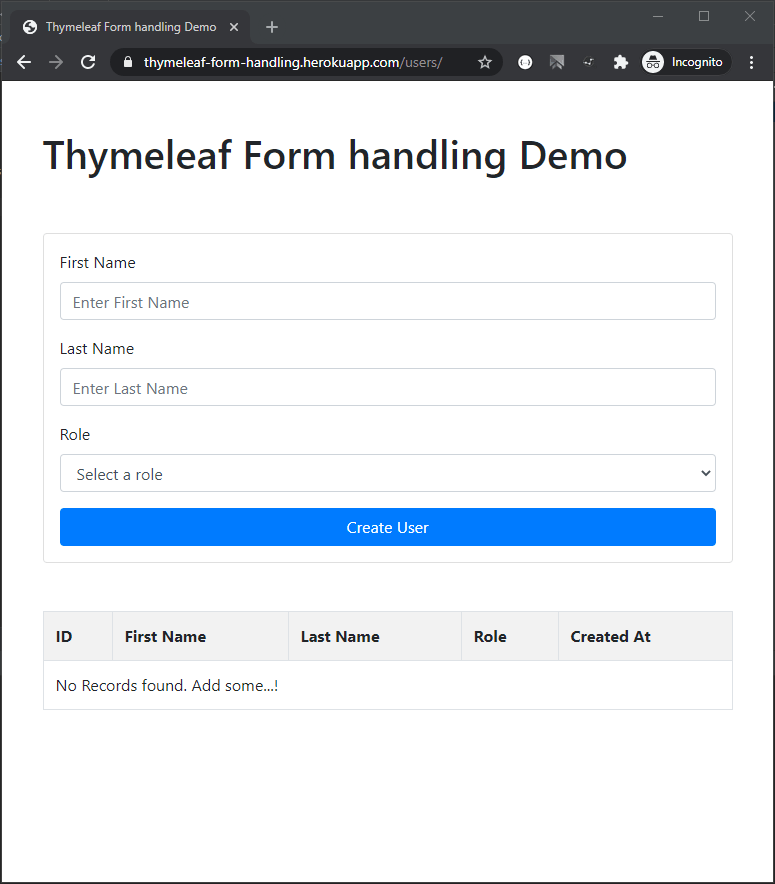
With the stats variable, you get the following aspects of a given list. They are,
Stat variable | Description |
---|---|
index | – The current index of the iteration, starting with 0. |
count | – The current index of the iteration, starting with 1. |
size | – The total amount of elements in the list. |
current | – The current element in the iteration. |
odd | – A boolean that is true if the current element is in an odd index of the list. |
even | – A boolean that is true if the current element is in an even index of the list. |
first | – A boolean that is true if the element in the iteration is in the first position of the list. |
last | – A boolean that is true if the element in the iteration is in the last position of the list. |
Good thing about thymeleaf is that if you don’t ask for a stats variable yourself, it will create one for you silently. For example, you can access the stat variable even without defining one.
<tr th:each="user : ${userList}">
<td class="align-middle" th:text="${userStat.count}"></td>
<td class="align-middle" th:text="${user.id}"></td>
<td class="align-middle" th:text="${user.firstName}"></td>
<td class="align-middle" th:text="${user.lastName}"></td>
</tr>
Code language: HTML, XML (xml)
As you notice, thymeleaf supplies a userStat
variable for you to make use of. The naming convention would be ** whatever the objectName + Stat**.
Summary
To summarize, We learned how to loop through lists using thymeleaf th:each attribute. If you want to learn more about thymeleaf, check out our post on how to build a CRUD web application using thymeleaf.
If you want examples of looping through lists in thymeleaf, checkout this github link.
You may also be interested in the following write-ups.
Hi! I’m having trouble sending a list of a node’s children ids to the spring controller using thymeleaf. Can you please check out this stack overflow ticket? Thank you!
https://stackoverflow.com/questions/69113957/how-do-you-send-a-list-of-a-nodes-children-ids-using-thymeleaf
I commented back with a few suggestions. Hope that helps.