Understanding Lazy Initialization in Spring Boot
Starting with Spring Boot 2.2, spring beans can be lazy. That is, You could create beans as and when required. Let’s see how to implement lazy initialization with Spring Boot.
What is lazy initialization?
By default, Spring Framework creates and injects beans and it’s dependencies at the time of context creation or refresh. But with lazy mode, We could initialize beans as and when they are needed. Spring can do this through proxy objects as placeholder beans. When the a call happens to these proxies, Spring can detect and create an appropriate bean for them.
How to enable Lazy Initialization?
The concept of lazy bean loading is not new for Spring framework. But Spring Boot 2.2 just makes it easier for you. To begin with, There are a couple of ways to enable lazy initialization. They are,
- Using properties file.
- Using built-in methods from
SpringApplication
orSpringApplicationBuilder
.
We shall go through each of these approaches one by one.
Using application properties
This approach requires a newly introduced spring.main.lazy-initialization
property entry. When set to true
, beans in the application will be lazy loaded.
spring.main.lazy-initialization=true
Code language: Properties (properties)
If you are using YAML, then you should use the following configuration.
spring:
main:
lazy-initialization: true
Code language: YAML (yaml)
Using built-in Spring Application methods
You could also set the lazyInitialization
flag in the SpringApplication
object to enable lazy init. And you could do that in two ways.
By creating the SpringApplication
object and setting the flag directly.
@SpringBootApplication
public class SpringBootLazyInitApplication {
public static void main(String[] args) {
SpringApplication application = new SpringApplication(SpringBootLazyInitApplication.class);
application.setLazyInitialization(true);
application.run(args);
}
}
Code language: JavaScript (javascript)
You could also set the lazy init flag while using SpringApplicationBuilder
.
@SpringBootApplication
public class SpringBootLazyInitApplication {
public static void main(String[] args) {
new SpringApplicationBuilder()
.main(SpringBootLazyInitApplication.class)
.lazyInitialization(true)
.build()
.run(args);
}
}
Code language: Java (java)
Testing the lazy initialization in Spring Boot.
To test the behaviour I created a bean that deliberately takes 10 seconds to create.
@Service
public class HelloService {
public HelloService() {
try {
Thread.sleep(10 * 1000);
} catch (InterruptedException ignored) {
}
}
public String hello() {
return "Hello There...!";
}
}
Code language: Java (java)
Without lazy, the spring Boot application should take a while to startup. let’s test this theory.

Let’s enable lazy initialize and restart out spring boot application.

As you could compare, The application starts faster this way. This is because the application didn’t need HelloService
Yet. So the constructor was never called to create the bean.
Advantages of Lazy initialization
There are various situations where lazy init is helpful.
- Lazy loading can reduce startup times as few classes are loaded fewer beans are created.
- Application startup is a CPU intensive task. By creating beans as and when needed, You are spreading the load over time.
- Faster startup times are useful in
Docker
andKubernetes
platforms.
Pitfalls of Lazy initialization
Even though the lazy loading may seem awesome, There are some underlying risks.
- Beans creation related errors may only be found at the time of loading the bean. Without lazy loading, we could identify them at the startup itself.
- Failures like no class def found errors, OOM errors, and failures due to misconfiguration would go unnoticed at the startup time.
- The initial requests may take more time now as the application will have to create the beans.
In web applications specifically, The first few requests may be slow. We could demonstrate this behavior by calling our HelloController
.
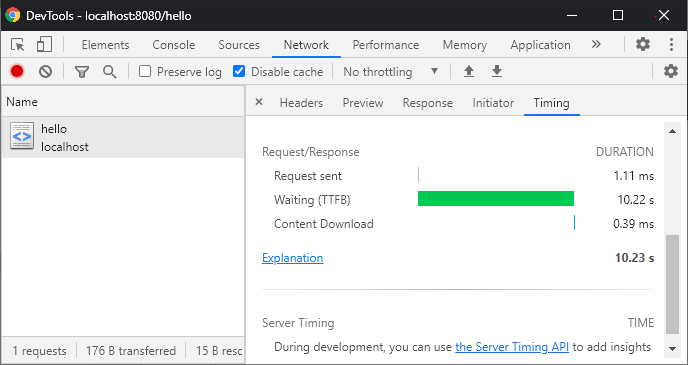
As you see in the screenshot, the request took 10+ seconds as HelloService initialized only after it was required. You could test this API at the demo given at the end of this article.
When to use Lazy Initialization?
Lazy initialization is helpful when the application is small. It also has place where components that has lower probability of being used. For example, applications with async communication doesn’t need to worry about the intial performance degrade. As long as your application is up and picking up the messages, you are good to go.
Developers could set this flag while writing the components as they only are writing and testing a specific component or two. This should ideally reduce the time reloading the application after every code change.
Summary
When used wisely, This feature may be an overpowered offering from Spring Boot yet. Also, you could checkout this sample project from this GitHub repository.
If you liked this article then You may also find the following articles relevant and interesting.