Send HTML emails with FreeMarker Templates – Spring Boot
Let’s learn how to send HTML emails from Spring Boot using FreeMarker template engine.
Background
Apache FreeMarker is a template engine that helps to create dynamic HTML content. This ability makes it suitable for generating emails based on HTML templates.
To demonstrate this, We are going to create an email service using Freemarker and Spring Boot.
Spring Boot Dependencies for Javamail And FreeMarker
Spring boot provides Starter dependencies for both javamail and Freemarker Template engine. We just have to add them to our pom.xml file.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
Code language: HTML, XML (xml)
The above dependencies will auto configure a FreeMarker template engine and a JavaMailSender object for us to send email.
Configuring JavaMailSender
The spring boot starter for email requires configuration properties to set up the SMTP server connection. For example, The following connects to GMAIL as an SMTP server and my google account as credentials.
spring.mail.username=[email protected]
spring.mail.password=*************
spring.mail.host=smtp.gmail.com
spring.mail.port=587
spring.mail.protocol=smtp
spring.mail.properties.mail.smtp.auth=true
spring.mail.properties.mail.smtp.starttls.enable=true
Code language: Properties (properties)
I’m using GMAIL for these settings. If you are developing for your organization, You should check with your administrator for these settings.
Also, GMAIL doesn’t allow Java programs to send emails by default. To enable it, you need to allow access to insecure apps.
Creating a Freemarker Email Template
Create your email.ftlh file under src/main/resources/templates as shown below.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Spring Boot Email using FreeMarker</title>
</head>
<body>
<div style="margin-top: 10px">Greetings, ${user.name}</div>
<div>Your username is <b>${user.username}</b></div>
<br/>
<div> Have a nice day..!</div>
</body>
</html>
Code language: HTML, XML (xml)
Here, the freemarker expressions are ${…}. Next, we need to write an Email Service that utilizes this template file. As you see, the expressions can be placed anywhere. That may be an advantage for some of us. But when it comes to maintaining these templates for the long run, Thymeleaf templates outlive freemarker.
FreeMarker Email Service
We are going to write an email service that does two things. One is to generate dynamic email content using Freemarker. Second, the mail sending logic itself. Let’s break down these steps a bit further.
The autoconfiguration creates a freemarker.template.Configuration bean. This bean will help us resolve to a Template using the file name. Once you have a template object, you can call the process method to get the generated content.
The model itself can be any object. For our case, We are using a HashMap so that we can refer to each entry by name.
@Service
public class EmailService {
final Configuration configuration;
final JavaMailSender javaMailSender;
public EmailService(Configuration configuration, JavaMailSender javaMailSender) {
this.configuration = configuration;
this.javaMailSender = javaMailSender;
}
public void sendEmail(UserInfo user) throws MessagingException, IOException, TemplateException {
MimeMessage mimeMessage = javaMailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(mimeMessage);
helper.setSubject("Welcome To SpringHow.com");
helper.setTo(user.getEmail());
String emailContent = getEmailContent(user);
helper.setText(emailContent, true);
javaMailSender.send(mimeMessage);
}
String getEmailContent(UserInfo user) throws IOException, TemplateException {
StringWriter stringWriter = new StringWriter();
Map<String, Object> model = new HashMap<>();
model.put("user", user);
configuration.getTemplate("email.ftlh").process(model, stringWriter);
return stringWriter.getBuffer().toString();
}
}
Code language: Java (java)
To let spring boot email sender know that we are using HTML content, set the HTML flag to true on helper.setText.
A controller to test the Service
Finally, to trigger the email, We need to write an API endpoint. In our case the template is for user registration. So let’s write a register controller.
public class UserInfo {
private String username;
private String name;
private String email;
//getter & setter
}
Code language: Java (java)
@RequestMapping(method = RequestMethod.POST,path = "/register")
@ResponseBody
public String register(@RequestBody UserInfo userInfo) throws Exception {
emailService.sendEmail(userInfo);
return "Email Sent..!";
}
Code language: Java (java)
Testing Email Service
To test our Spring Boot Email Service using Freemarker, We just need to send a request to our register API. So, Let’s do that with from Postman.
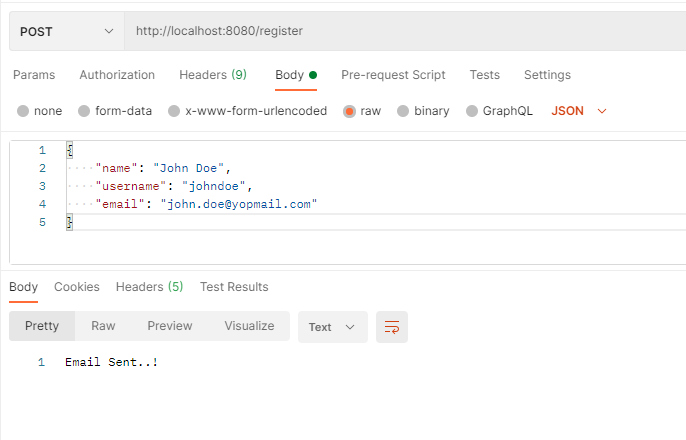
In this case, I’m using a disposable email to test if the email works properly. As you see in the below screenshot, The message indeed gets delivered properly.
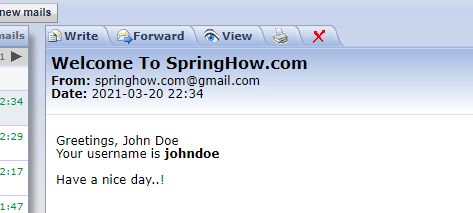
Conclusion
In this post, We learned how to send HTML emails using the Freemarker template engine with an example. If you are interested, you can also send emails using thymeleaf. Also, you can give a read on the following articles if interested.