Introduction to FreeMarker Templates with Spring Boot
FreeMarker is a java based template engine which has rich support in Spring Boot. In this tutorial, we will learn how to use Apache FreeMarker as a template engine for Spring MVC with an example.
Introduction
Spring Boot provides out of the box support to four major template engines. Out of these four, FreeMarker has been the consistent performer. With this template engine, you can create dynamic MVC views, And that is what we are going to learn today.
FreeMarker Dependencies
To add support to Freemarker Templates in a Spring Boot application, You should add the following starter dependency.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
</dependency>
Code language: HTML, XML (xml)
This dependency provides a FreeMarkerServletWebConfiguration which sets up the MVC related settings.
Freemarker Template Files
Spring boot looks for the The template files under classpath:/templates/. And for the file extension, you should name the files with .ftlh suffix since Spring Boot 2.2. If you plan on changing the location or the file extension, you should use the following configurations.
spring.freemarker.suffix=.ftlh
spring.freemarker.template-loader-path=classpath:/templates/
Code language: Properties (properties)
To see the freemarker in action, You need to create a template file and map it to a controller method. Let’s see how to do that.
Sample Template
Take a look at this hello.ftlh file. This file takes a template variable called name and prints it in the output.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>FreeMarker Spring Boot Example</title>
</head>
<body>
<h1>Hello ${name}!</h1>
</body>
</html>
Code language: HTML, XML (xml)
Let’s write a controller method for it.
Hello Controller
Spring Boot controllers are all similar when it comes to template engines, Only the implementing engine changes. For example, I’m using a similar controller that we used in the thymeleaf template engine tutorial.
@RequestMapping(method = RequestMethod.GET, path = "/hello")
public String hello(@RequestParam(name = "name") String name, Model model) {
model.addAttribute("name", name);
return "hello";
}
Code language: Java (java)
Note the highlighted line. It should match the template file that we created earlier. Also note that I’m taking a request parameter and passing it as a model attribute. The Spring MVC will take care of passing this model attribute to the freemarker template engine.
Results
If we have done all of this right, You can see the “Hello world!” message at the url http://localhost:8080/hello?name=world
.
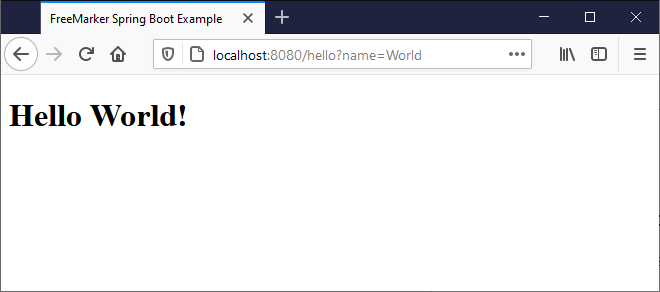
Conclusion
In this post, we learned how to use free marker template files with Spring Boot application using MVC. If you liked this article, please give a read on the following topics.
If you want to play with this example, I have a demo project available at this GitHub repository.